Sveiki,
gal kas galetu paaiskinti kaip istaisyti sitas klaidas.Noriu isdeti PasswordChanger NPC ir Casino NPC.
Problemos kaip matote zemiau nurodytos nuotraukose.
Naudoju L2jFrozen naujausia rev.
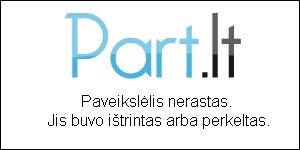

1.Kodas
/* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2, or (at your option)
* any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA
* 02111-1307, USA.
*
* http://www.gnu.org/copyleft/gpl.html
*/
package com.l2jfrozen.gameserver.model.actor.instance;
import com.l2jfrozen.crypt.Base64;
import com.l2jfrozen.gameserver.ai.CtrlIntention;
import com.l2jfrozen.gameserver.network.serverpackets.ActionFailed;
import com.l2jfrozen.gameserver.network.serverpackets.LeaveWorld;
import com.l2jfrozen.gameserver.network.serverpackets.MyTargetSelected;
import com.l2jfrozen.gameserver.network.serverpackets.NpcHtmlMessage;
import com.l2jfrozen.gameserver.network.serverpackets.ValidateLocation;
import com.l2jfrozen.gameserver.templates.L2NpcTemplate;
import com.l2jfrozen.util.database.L2DatabaseFactory;
import java.security.MessageDigest;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.StringTokenizer;
import javolution.text.TextBuilder;
public class L2PasswordChangerInstance extends L2FolkInstance
{
public L2PasswordChangerInstance(int objectId, L2NpcTemplate template)
{
super(objectId, template);
}
public void onBypassFeedback(L2PcInstance player, String command)
{
if (command.startsWith("change_password"))
{
StringTokenizer st = new StringTokenizer(command);
st.nextToken();
String currPass = null;
String newPass = null;
String repeatNewPass = null;
try
{
if (st.hasMoreTokens())
{
currPass = st.nextToken();
newPass = st.nextToken();
repeatNewPass = st.nextToken();
}
else
{
player.sendMessage("Please fill in all the blanks before requesting for a password change.");
return;
}
changePassword(currPass, newPass, repeatNewPass, player);
}
catch (StringIndexOutOfBoundsException e)
{
}
}
}
@Override
public void onAction(L2PcInstance player)
{
if (!canTarget(player)) {
return;
}
if (this != player.getTarget())
{
player.setTarget(this);
player.sendPacket(new MyTargetSelected(getObjectId(), 0));
player.sendPacket(new ValidateLocation(this));
}
else if (!canInteract(player))
{
player.getAI().setIntention(CtrlIntention.AI_INTENTION_INTERACT, this);
}
else
{
showHtmlWindow(player);
}
player.sendPacket(new ActionFailed());
}
private void showHtmlWindow(L2PcInstance activeChar)
{
NpcHtmlMessage nhm = new NpcHtmlMessage(5);
TextBuilder replyMSG = new TextBuilder("");
replyMSG.append("<html><title>L2-PvP Account Manager</title>");
replyMSG.append("<body><center>");
replyMSG.append("To change your password:<br1> First fill in your current password and then your new!</font><br>");
replyMSG.append("Current Password: <edit var=\"cur\" width=100 height=15><br>");
replyMSG.append("New Password: <edit var=\"new\" width=100 height=15><br>");
replyMSG.append("Repeat New Password: <edit var=\"repeatnew\" width=100 height=15><br><br>");
replyMSG.append("<button value=\"Change Password\" action=\"bypass -h npc_" + getObjectId() + "_change_password $cur $new $repeatnew\" width=204 height=20 back=\"sek.cbui75\" fore=\"sek.cbui75\">");
replyMSG.append("</center></body></html>");
nhm.setHtml(replyMSG.toString());
activeChar.sendPacket(nhm);
activeChar.sendPacket(new ActionFailed());
}
public static boolean changePassword(String currPass, String newPass, String repeatNewPass, L2PcInstance activeChar)
{
if (newPass.length() < 5)
{
activeChar.sendMessage("The new password is too short!");
return false;
}
if (newPass.length() > 20)
{
activeChar.sendMessage("The new password is too long!");
return false;
}
if (!newPass.equals(repeatNewPass))
{
activeChar.sendMessage("Repeated password doesn't match the new password.");
return false;
}
Connection con = null;
String password = null;
try
{
MessageDigest md = MessageDigest.getInstance("SHA");
byte[] raw = currPass.getBytes("UTF-8");
raw = md.digest(raw);
String currPassEncoded = Base64.encodeBytes(raw);
con = L2DatabaseFactory.getInstance().getConnection();
PreparedStatement statement = con.prepareStatement("SELECT password FROM accounts WHERE login=?");
statement.setString(1, activeChar.getAccountName());
ResultSet rset = statement.executeQuery();
while (rset.next())
{
password = rset.getString("password");
}
rset.close();
statement.close();
byte[] password2 =
null;
if (currPassEncoded.equals(password))
{
password2 = newPass.getBytes("UTF-8");
password2 = md.digest(password2);
PreparedStatement statement2 = con.prepareStatement("UPDATE accounts SET password=? WHERE login=?");
statement2.setString(1, Base64.encodeBytes(password2));
statement2.setString(2, activeChar.getAccountName());
statement2.executeUpdate();
statement2.close();
activeChar.sendMessage("Congratulations! Your password has been changed succesfully. You will now be disconnected for security reasons. Please login again!");
try
{
Thread.sleep(3000L);
}
catch (Exception e)
{
}
activeChar.deleteMe();
activeChar.sendPacket(new LeaveWorld());
}
else
{
activeChar.sendMessage("The current password you've inserted is incorrect! Please try again!");
return password2 != null;
}
}
catch (Exception e)
{
_log.warning("could not update the password of account: " + activeChar.getAccountName());
}
finally
{
try
{
if (con != null)
con.close();
}
catch (SQLException e)
{
_log.warning("Failed to close database connection!");
}
}
return true;
}
}
2. Kodas
package com.l2jfrozen.gameserver.model.actor.instance;
import com.l2jfrozen.gameserver.ai.CtrlIntention;
import com.l2jfrozen.gameserver.network.serverpackets.NpcHtmlMessage;
import com.l2jfrozen.gameserver.network.serverpackets.SetupGauge;
import com.l2jfrozen.gameserver.network.serverpackets.SocialAction;
import com.l2jfrozen.gameserver.templates.L2NpcTemplate;
import com.l2jfrozen.gameserver.thread.ThreadPoolManager;
import com.l2jfrozen.util.random.Rnd;
import java.util.NoSuchElementException;
import java.util.StringTokenizer;
import javolution.text.TextBuilder;
public class L2CasinoInstance extends L2NpcInstance
{
public L2CasinoInstance(int objectId, L2NpcTemplate template)
{
super(objectId, template);
}
public void onBypassFeedback(L2PcInstance player, String command)
{
int am-beep-t = 0;
if (command.startsWith("play1"))
{
StringTokenizer st = new StringTokenizer(command);
try
{
st.nextToken();
am-beep-t = Integer.parseInt(st.nextToken());
}
catch (NoSuchElementException nse)
{
}
Casino1(player, am-beep-t);
}
}
public static void displayCongrats(L2PcInstance player)
{
player.broadcastPacket(new SocialAction(player.getObjectId(), 3));
player.sendMessage("Congratulations You Won!");
}
public static void displayCongrats2(L2PcInstance player)
{
player.sendMessage("You lost!");
}
public void showChatWindow(L2PcInstance player, int val)
{
NpcHtmlMessage msg = new NpcHtmlMessage(getObjectId());
msg.setHtml(casinoWindow(player));
msg.replace("%objectId%", String.valueOf(getObjectId()));
player.sendPacket(msg);
}
private String casinoWindow(L2PcInstance player)
{
TextBuilder tb = new TextBuilder();
tb.append("<html><title>L2 InStadia Casino Manager</title><body>");
tb.append("<center><img src=\"L2UI_CH3.onscrmsg_pattern01_1\" width=295 height=32><br><br>");
tb.append("<font color=\"3b8d8d\">Chance to win : 50%</font><br>");
tb.append("<img src=\"L2UI.SquareGray\" width=\"280\" height=\"1\"><br>");
tb.append("<tr><td><font color=\"e0d6b9\">Double or Nothing </font></td></tr><br>");
tb.append("<img src=\"L2UI.SquareGray\" width=\"280\" height=\"1\"></center><br>");
tb.append("<center>");
tb.append("<font color=\"e0d6b9\">Place your bet: Vote Items!</font>");
tb.append("</center>");
tb.append("<center><img src=\"L2UI.SquareGray\" width=\"280\" height=\"1\"></center><br>");
tb.append("<br>");
tb.append("<center>");
tb.append("<tr>");
tb.append("<td align=center><edit var=\"qbox\" width=120 height=15><br></td> <td align=right></td>");
tb.append("<td align=center><button value=\"Bet\" action=\"bypass -h npc_%objectId%_play1 $qbox\" width=204 height=20 back=\"sek.cbui75\" fore=\"sek.cbui75\"></td>");
tb.append("</tr>");
tb.append("</center>");
tb.append("<center><img src=\"L2UI_CH3.onscrmsg_pattern01_2\" width=295 height=32><br></center>");
tb.append("</body></html>");
return tb.toString();
}
public static void Casino1(L2PcInstance player, int am-beep-t)
{
int unstuckTimer = 100;
player.setTarget(player);
player.getAI().setIntention(CtrlIntention.AI_INTENTION_IDLE);
player.disableAllSkills();
SetupGauge sg = new SetupGauge(0, unstuckTimer);
player.sendPacket(sg);
Casino1 ef = new Casino1(player, am-beep-t);
player.setSkillCast(ThreadPoolManager.getInstance().scheduleGeneral(ef, unstuckTimer));
}
static class Casino1 implements Runnable {
private L2PcInstance _player;
private int _am-beep-t;
Casino1(L2PcInstance player, int am-beep-t) {
this._am-beep-t = am-beep-t;
this._player = player;
}
public void run() {
if (this._player.isDead())
{
return;
}
this._player.setIsIn7sDungeon(false);
this._player.enableAllSkills();
int chance = Rnd.get(3);
if (this._player.getInventory().getInventoryItemCount(11001, 0) >= this._am-beep-t)
{
if (chance == 0)
{
L2CasinoInstance.displayCongrats(this._player);
this._player.addItem("Gift", 11001, this._am-beep-t, this._player, true);
this._player.broadcastUserInfo();
}
if (chance == 1)
{
L2CasinoInstance.displayCongrats2(this._player);
this._player.destroyItemByItemId("Consume", 11001, this._am-beep-t, this._player, true);
this._player.broadcastUserInfo();
}
if (chance == 2)
{
L2CasinoInstance.displayCongrats2(this._player);
this._player.destroyItemByItemId("Consume", 11001, this._am-beep-t, this._player, true);
this._player.broadcastUserInfo();
}
}
else
{
this._player.sendMessage("You do not have enough Vote Items!");
}
}
}
}
Paskutinį kartą redagavo: 20 Lapkričio 2017 - 16:31